to provide advanced features such as zero-config time-travel debugging and state snapshot export / import.
Let's start with a simple Vue counter app: What is a "State Management Pattern"?
new Vue({
// state
data () {
return {
count: 0
}
},
// view
template: `
<div>{{ count }}</div>
`,
// actions
methods: {
increment () {
this.count++
}
}
})
- The state, which is the source of truth that drives our app;
- The view, which is just a declarative mapping of the state;
- The actions, which are the possible ways the state could change in reaction to user inputs from the view.
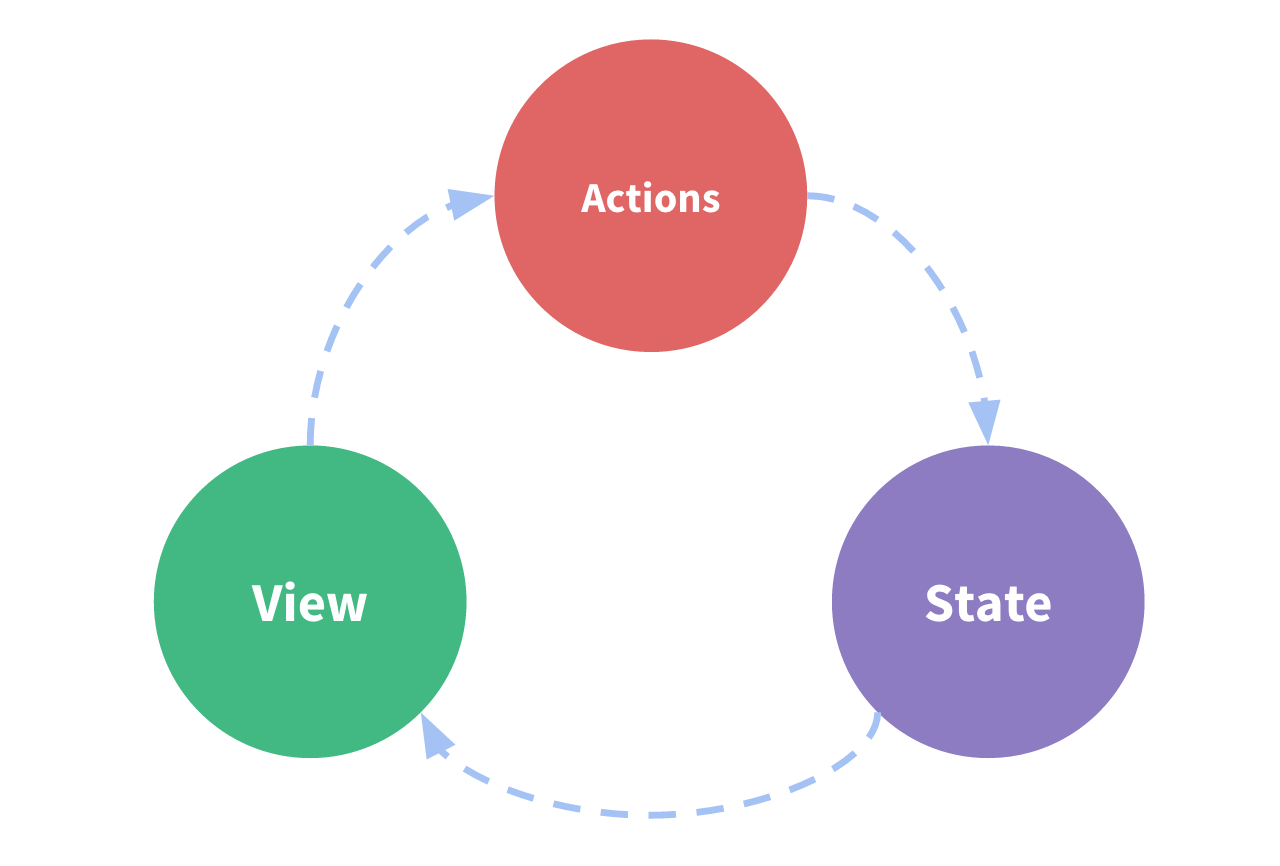
- Multiple views may depend on the same piece of state.
- Actions from different views may need to mutate the same piece of state.
So why don't we extract the shared state out of the components, and manage it in a global singleton? With this, our component tree becomes a big "view", and any component can access the state or trigger actions, no matter where they are in the tree!
In addition, by defining and separating the concepts involved in state management and enforcing certain rules, we also give our code more structure and maintainability.
This is the basic idea behind Vuex, inspired by Flux
, Redux and The Elm Architecture . Unlike the other patterns, Vuex is also a library implementation tailored specifically for Vue.js to take advantage of its granular reactivity system for efficient updates.
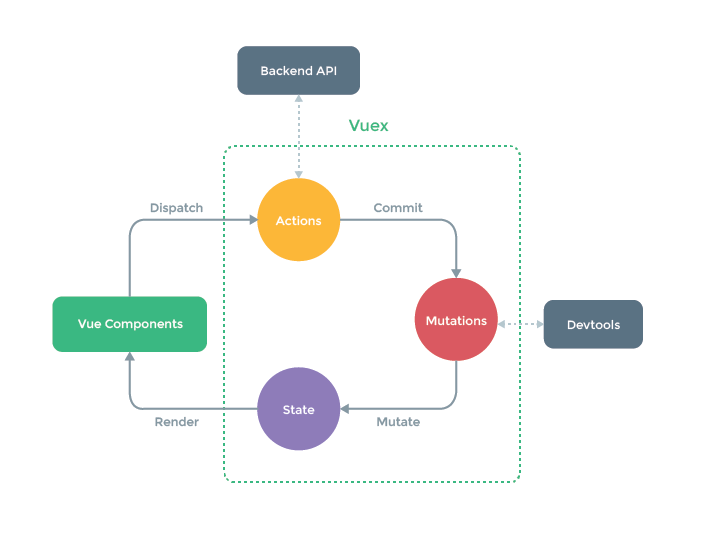
Although Vuex helps us deal with shared state management, it also comes with the cost of more concepts and boilerplate. It's a trade-off between short term and long term productivity. When Should I Use It?
If you've never built a large-scale SPA and jump right into Vuex, it may feel verbose and daunting. That's perfectly normal - if your app is simple, you will most likely be fine without Vuex. A simple store pattern
may be all you need. But if you are building a medium-to-large-scale SPA, chances are you have run into situations that make you think about how to better handle state outside of your Vue components, and Vuex will be the natural next step for you. There's a good quote from Dan Abramov, the author of Redux:
Flux libraries are like glasses: you’ll know when you need them.
Installation
Direct Download / CDN
https://unpkg.com/vuexUnpkg.com provides NPM-based CDN links. The above link will always point to the latest release on NPM. You can also use a specific version/tag via URLs like
https://unpkg.com/vuex@2.0.0
.
Include
vuex
after Vue and it will install itself automatically:<script src="/path/to/vue.js"></script>
<script src="/path/to/vuex.js"></script>
NPM
npm install vuex --save
Yarn
yarn add vuex
Vue.use()
:import Vue from 'vue'
import Vuex from 'vuex'
Vue.use(Vuex)
Vuex requires PromisePromise
. If your supporting browsers do not implement Promise (e.g. IE), you can use a polyfill library, such as es6-promise .
You can include it via CDN:
<script src="https://cdn.jsdelivr.net/npm/es6-promise@4/dist/es6-promise.auto.js"></script>
window.Promise
will be available automatically.If you prefer using a package manager such as NPM or Yarn, install it with the following commands:
npm install es6-promise --save # NPM
yarn add es6-promise # Yarn
import 'es6-promise/auto'
You will have to clone directly from GitHub and build Dev Build
vuex
yourself if you want to use the latest dev build.git clone https://github.com/vuejs/vuex.git node_modules/vuex
cd node_modules/vuex
npm install
npm run build
Getting Started
At the center of every Vuex application is the store. A "store" is basically a container that holds your application state. There are two things that make a Vuex store different from a plain global object:- Vuex stores are reactive. When Vue components retrieve state from it, they will reactively and efficiently update if the store's state changes.
- You cannot directly mutate the store's state. The only way to change a store's state is by explicitly committing mutations. This ensures every state change leaves a track-able record, and enables tooling that helps us better understand our applications.
The Simplest Store
NOTE: We will be using ES2015 syntax for code examples for the rest of the docs. If you haven't picked it up, you should
!After installing Vuex, let's create a store. It is pretty straightforward - just provide an initial state object, and some mutations:
// Make sure to call Vue.use(Vuex) first if using a module system
const store = new Vuex.Store({
state: {
count: 0
},
mutations: {
increment (state) {
state.count++
}
}
})
store.state
, and trigger a state change with the store.commit
method:store.commit('increment')
console.log(store.state.count) // -> 1
store.state.count
directly, is because we want to explicitly track it. This simple
convention makes your intention more explicit, so that you can reason
about state changes in your app better when reading the code. In
addition, this gives us the opportunity to implement tools that can log
every mutation, take state snapshots, or even perform time travel
debugging.Using store state in a component simply involves returning the state within a computed property, because the store state is reactive. Triggering changes simply means committing mutations in component methods.
Here's an example of the most basic Vuex counter app
.
Next, we will discuss each core concept in much finer details, starting with State.
No comments:
Post a Comment